Introduction
QR codes have become ubiquitous in digital communication, offering a quick way to link the physical and digital worlds. Python, with its simplicity and vast library ecosystem, provides an excellent toolkit for creating these codes. Whether you’re a marketer looking to add QR codes to promotional materials, a developer integrating scanning functionality into apps, or just curious about coding, this guide will walk you through generating QR codes using Python step by step.
Blog Summary
This blog will cover:
- What is a QR Code? – A brief overview of QR codes and their uses.
- Why Python for QR Codes? – Discussion on why Python is suitable for generating QR codes.
- Setting Up Your Environment – Instructions on preparing your Python environment for QR code generation.
- Step-by-Step Guide to Generating QR Codes – Detailed steps, including code examples.
- Customizing Your QR Codes – How to add logos, change colors, and alter sizes.
- Real-World Applications – Examples of how QR codes can be used in various projects.
- Troubleshooting Common Issues – Tips on solving typical problems you might encounter.
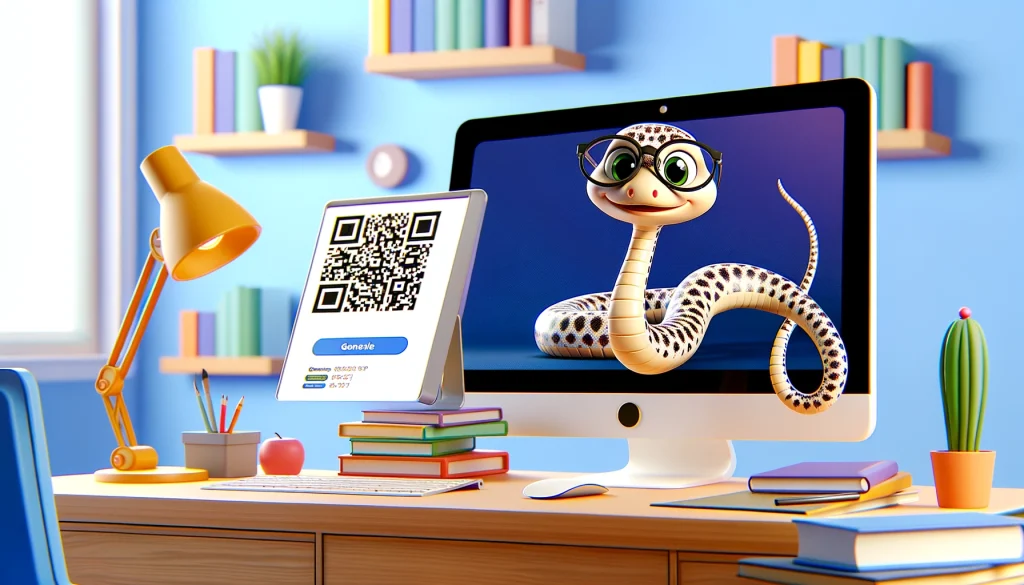
QR codes have surged in popularity as a bridge between physical and digital spaces, providing a swift means to transmit information ranging from URLs to complex business details. Python, known for its simplicity and robust library support, serves as an excellent platform for generating and experimenting with QR codes. In this guide, you’ll learn exactly how to create your own QR codes using Python, ideal for adding modern touches to your projects or streamlining user interactions.
What is a QR Code?
Quick Response (QR) codes are two-dimensional barcodes storing information both horizontally and vertically. They can encode various types of data, such as text, URLs, or even multimedia content, and are easily scannable by mobile devices, making them extremely useful for marketing, informational tags, and personal projects.
Why Python for QR Codes?
Python makes it easy to handle various data types and integrates seamlessly with several powerful libraries designed for image handling and code generation, including those needed for creating QR codes. Its straightforward syntax and vast community support simplify the learning curve for new developers and enhance productivity in project development.
Setting Up Your Environment
Before generating QR codes, ensure your Python environment is set up. Here’s how to prepare:
- Install Python: Make sure Python is installed on your computer. You can download it from python.org.
- Install Pip: Pip is Python’s package installer. It usually comes with Python, but you can check its installation by running
pip --version
in your command line. - Install Libraries: You’ll need the
qrcode
andPillow
libraries. Install them using pip:
pip install qrcode[pil]
Step-by-Step Guide to Generating QR Codes
Once your environment is ready, follow these steps to create your first QR code:
- Import the Library:
import qrcode
- Create QR Code Instance:
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
- Add Data to QR Code:
qr.add_data('https://www.example.com')
qr.make(fit=True)
- Generate and Save the Image:
img = qr.make_image(fill='black', back_color='white')
img.save('example_qr.png')
Customizing Your QR Codes
Customizing QR codes can enhance their appeal and functionality:
- Change Colors: Modify
fill
andback_color
inmake_image()
. - Add a Logo: Combine QR with an image using
Pillow
to embed logos. - Adjust Size: Manipulate
box_size
andborder
in the QRCode object.

Real-World Applications
QR codes can be used in numerous scenarios:
- Business Cards: Embed contact information.
- Promotional Materials: Link to special offers or event details.
- Operational Efficiencies: Track inventory or streamline operations.
Troubleshooting Common Issues
Here are quick fixes for typical problems:
- Poor Scanning: Increase the
border
size or adjust theerror_correction
level. - Data Overload: Opt for a higher
version
number to increase data capacity.
Further Resources
Explore more about QR codes with these resources:
By following this guide, you can integrate QR code functionalities into your Python projects, enhancing both the user experience and the effectiveness of your digital interactions. Happy coding!
Leave a response to this article by providing your insights, comments, or requests for future articles.
Share the articles with your friends and colleagues on social media